Alright, so let me walk you through something I was tinkering with recently. The idea kind of popped into my head, combining my tech side with a bit of downtime fun – crosswords.
I enjoy doing crosswords, you know, the paper kind sometimes. And often, especially in the tech-themed ones, you get clues related to software, old hardware, acronyms, that sort of thing. I thought, wouldn’t it be neat to have a little helper tool? Not something that gives you the answer straight up, but maybe something that could quickly check definitions or possibilities based on letters I already had, specifically for tech terms.
The Initial Idea
So, I pictured this little command-line thing. You’d type in a pattern, maybe some known letters, and specify it’s a ‘tech’ clue. My initial thought was to make it super flexible. Maybe I could have different search modes, like searching my own notes, searching a specific tech glossary file I have, or even trying different variations of an acronym.
This is where the ‘exec’ idea kinda snuck in. I was thinking, mainly in Python because it’s quick for scripting, how could I make it call different search functions based on user input? Like, user types `search_notes ‘pyhn’` and it runs the note-searching code, or `search_glossary ‘API’` and it runs the glossary code.
Trying the ‘Exec’ Approach
My first naive thought was, “Hey, maybe I can just build the function call as a string and use `exec` to run it!” It sounded simple on paper. Get the command, get the arguments, mash them together into a line of code like `search_notes(‘pyhn’)`, and then just `exec` it.
- I started scripting it out. Got the basic input reading done.
- Then I tried forming the command string. This already got a bit fiddly with quoting and making sure the arguments were passed correctly.
- Then I put in the `exec` call.
And yeah, it kinda worked for the first simple test. But almost immediately, alarm bells started ringing in my head.
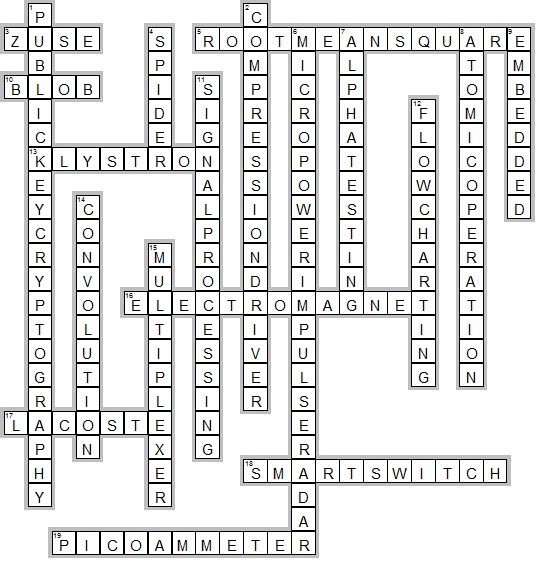
Why It Felt Wrong
Security, mostly. Even for a tiny personal tool, using `exec` with user input felt really dangerous. What if I accidentally typed something that broke the code structure? Or worse, what if the input somehow contained malicious commands? It just opens up a can of worms. If `exec` can run any code, it can run code that deletes files or does other bad stuff. That felt way too risky, even just running on my own machine.
Debugging was also looking like it would be a pain. If the dynamically generated code inside `exec` failed, tracing the error back would be harder than with regular function calls.
Switching Gears
I scrapped the `exec` idea pretty quickly. It was a classic case of reaching for a complex tool when a simple one would do. I went back and changed the approach:
- I created a dictionary mapping command names (like `”search_notes”`) to the actual Python functions (like `my_notes_search_function`).
- When the user types a command, I look up the command name in the dictionary.
- If it exists, I call the associated function directly, passing the user’s arguments to it.
This way felt much cleaner and safer. No arbitrary code execution. It’s just a straightforward lookup and function call. Much easier to read, debug, and sleep at night knowing it wasn’t a ticking time bomb.
The Outcome
The final tool is pretty basic. It does what I wanted – helps me quickly check tech terms when I’m stuck on a crossword. But the real takeaway was the process itself. Playing with `exec` reminded me why it’s generally avoided unless you really know what you’re doing and have carefully controlled the input. That little experiment reinforced the idea that simple, direct code is usually better than overly clever or complex solutions, especially when security is a concern.
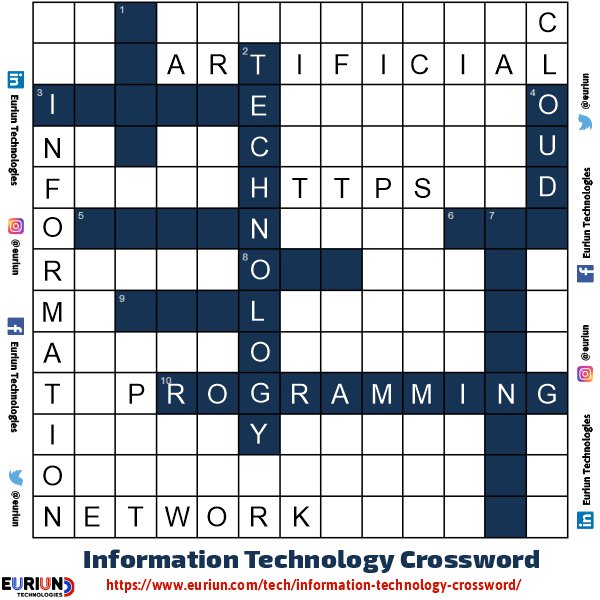
So yeah, that was my practice run trying to link dynamic execution with tech crosswords. An interesting thought experiment, but definitely sticking to safer methods for anything real.