Okay, so I’ve been messing around with this thing called Fritz AI for a while, and I finally got image prediction working. I wanted to share how I did it, ’cause it took me a bit to figure everything out.
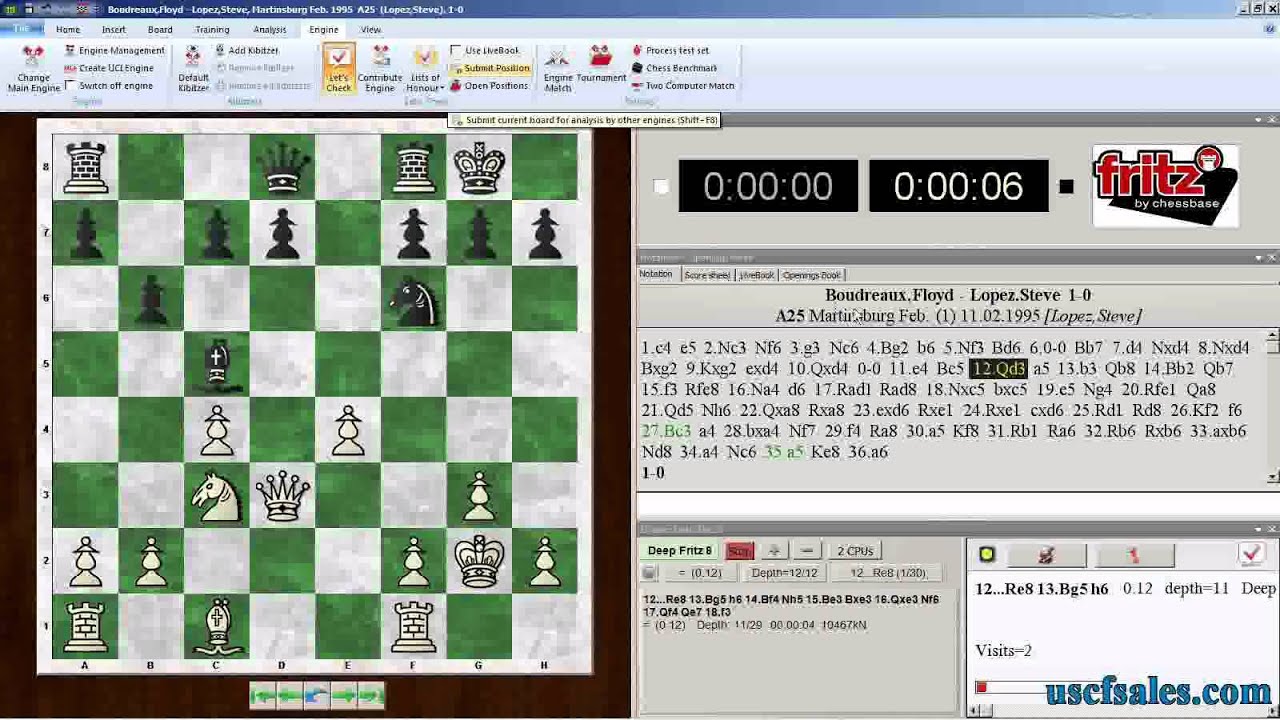
First, I went and signed up for an account on their website. It’s pretty straightforward, you know, the usual email and password stuff. Once I was in, I created a new project. They have all sorts of options, but I went straight for the “Image Labeling” one.
Next up, the model. I was playing around with a few custom models. I uploaded my datasets and had fritz trained it for me, cost me some credits though.
Getting the Code Right
This is where it got a little tricky for me. I’m not the best coder, so I copied their example code for Swift (I’m building an iOS app). There were a few things I had to change:
- My API Key: I had to replace the placeholder API key in their code with the one from my Fritz account dashboard. Don’t share this with anyone, by the way!
- Model ID: I also had to replace the default Model ID.
- Input Image: I was using a UIImage from my app, so I had to figure out how to feed that into the FritzVisionImage object. Turns out, it’s pretty easy, just create it directly from the UIImage.
- Getting the Prediction: The code shows how to get the top prediction, but I wanted to see all of them, with their confidence scores. So I added a loop to go through the results array and print everything out.
Testing It Out
After I made those changes, I ran the app on my phone. And…boom! It worked! The app was showing me the predicted labels for the images I gave it, along with how sure it was. It wasn’t perfect, some predictions are a bit off, but it’s pretty darn good for a start. I did have to play the min confidence to get the right predictions.
It felt great to finally see it working. I’m still experimenting with different images and tweaking the code, but it’s been a fun little project. If you’re thinking about trying out image prediction, Fritz seems like a decent option, especially if you’re not a machine learning expert (like me!).
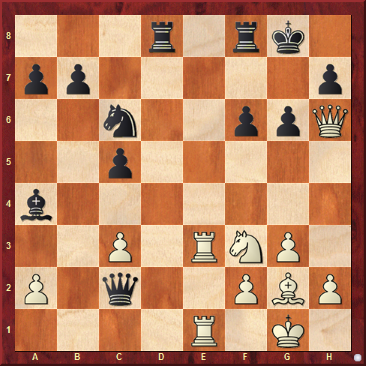
Just wanted to show the prediction code here:
swift
let visionImage = FritzVisionImage(image: myImage)
* = FritzVisionImageMetadata()
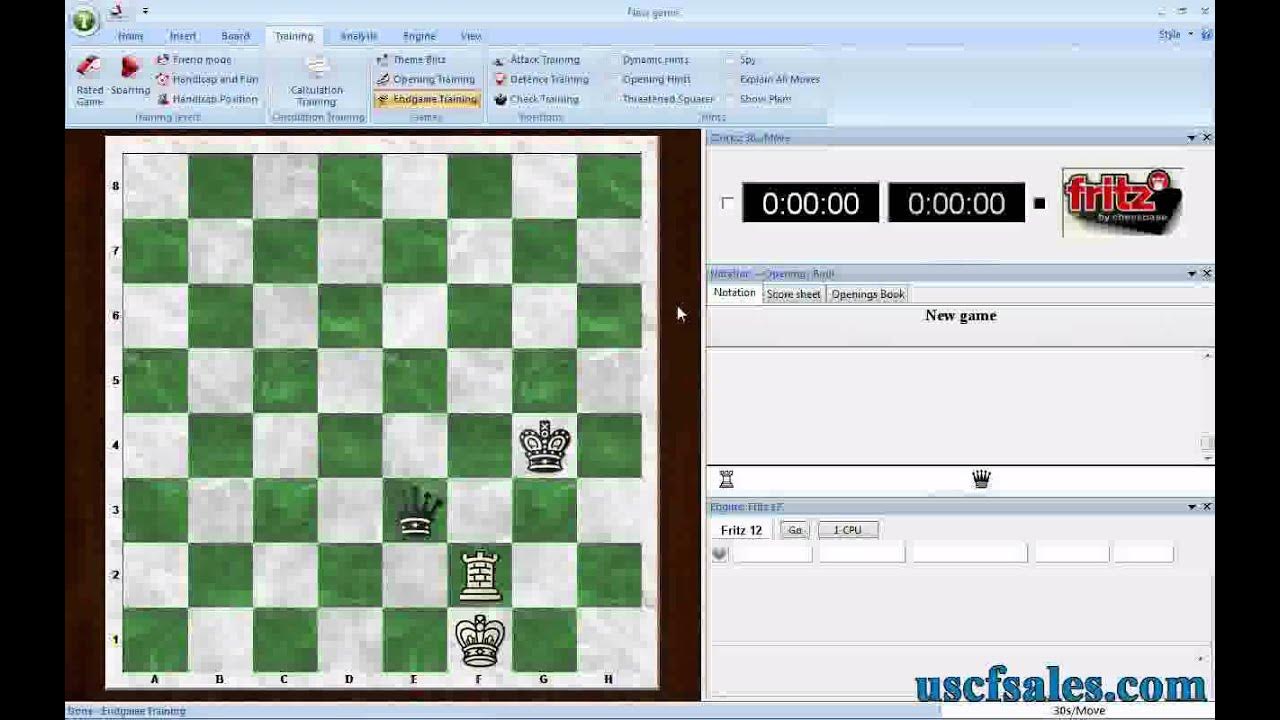
// the model ID here.
let predictor = FritzVisionImagePredictor(model: model, options: options)
*(visionImage, completion: { (labels, error) in
// Ensure there are no errors
if let error = error {
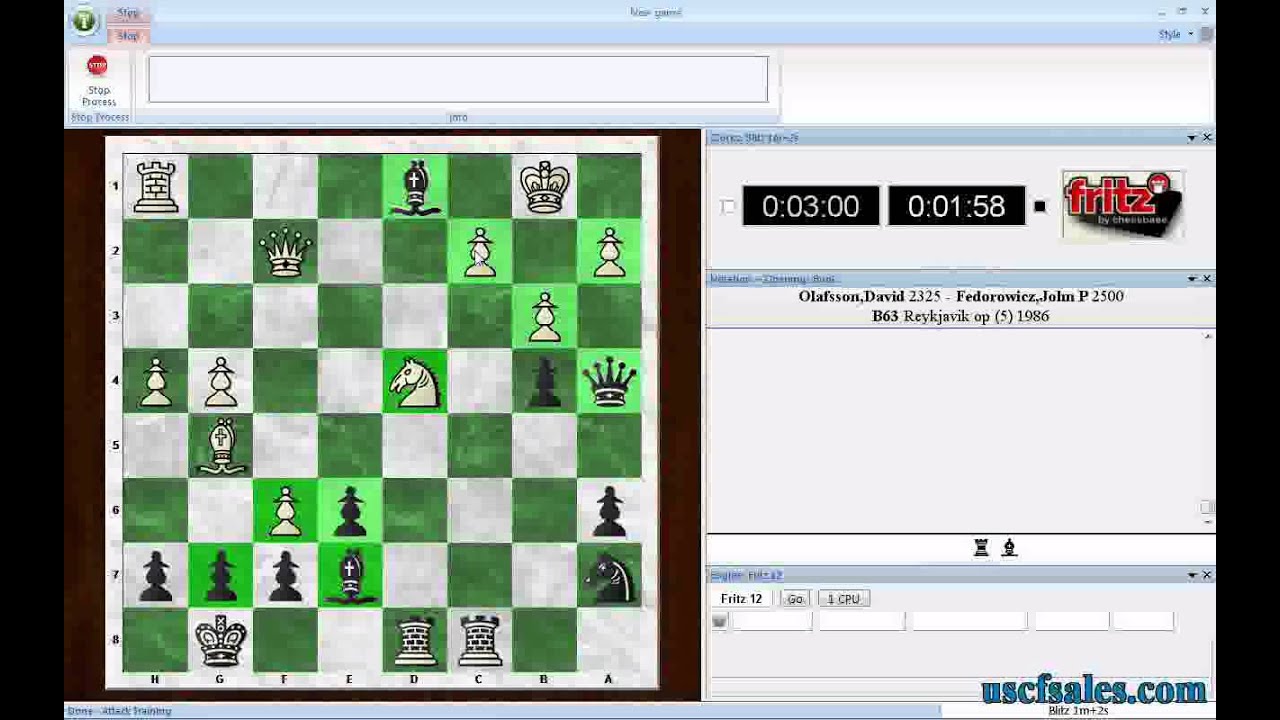
NSLog(“Prediction Error. (*)”)
return
// Ensure there are predictions
guard let labels = labels, * > 0 else {
NSLog(“No labels returned”)
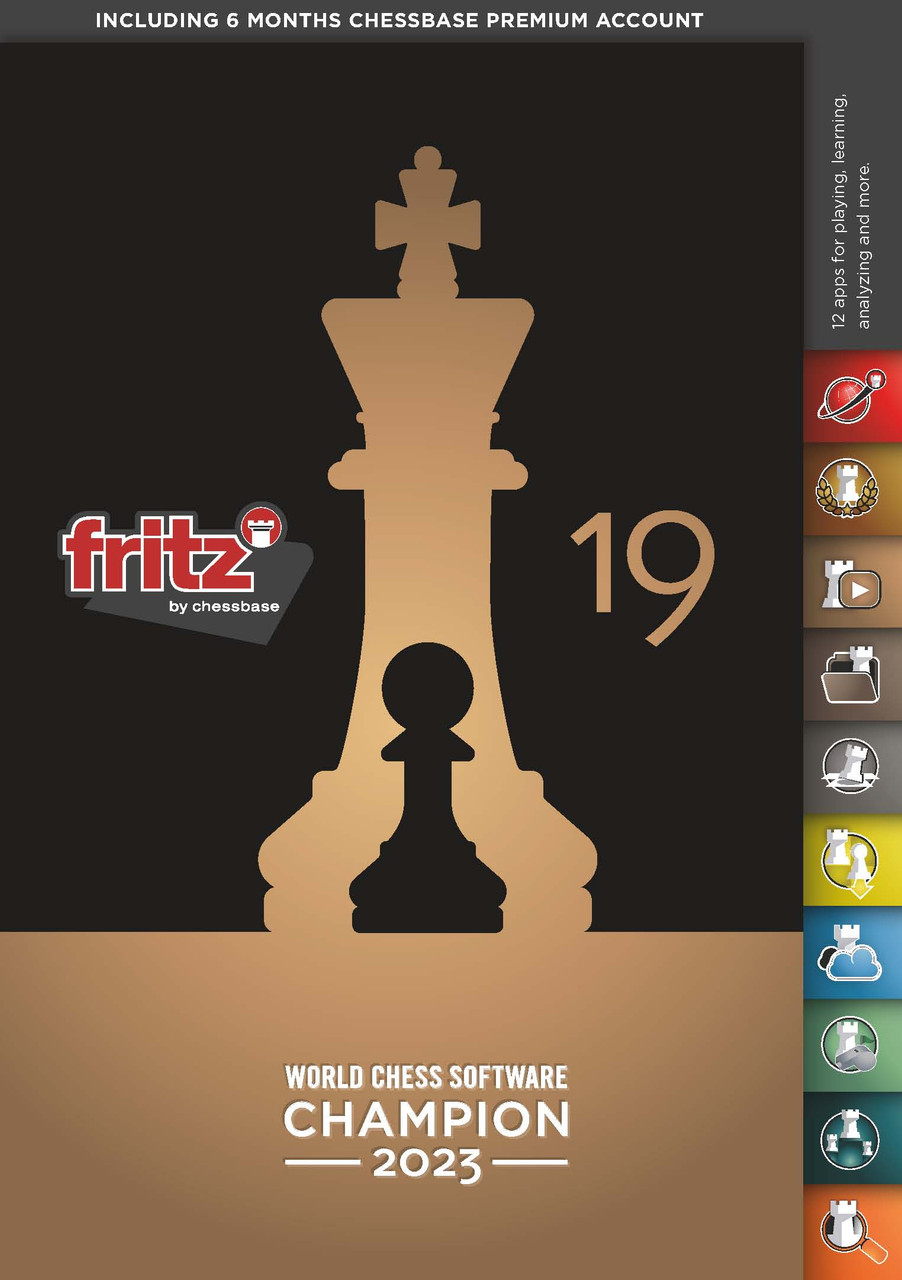
return
// Display the top prediction
for label in labels{
print(*)
print(*)
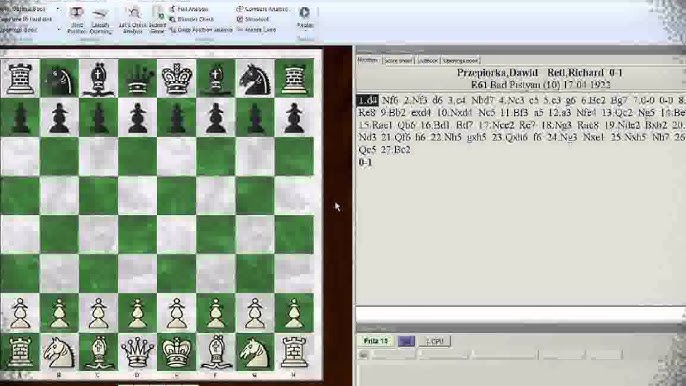
I had to learn about the options, turns out there are options class that I had to setup to fine tune my predictions.
swift
let options = FritzVisionLabelModelOptions()
* = 0.6
Hope this helps someone out there!