Alright, let’s talk about this crash logger thing I put together using FNV hashing. It wasn’t some grand plan, more like something born out of frustration.
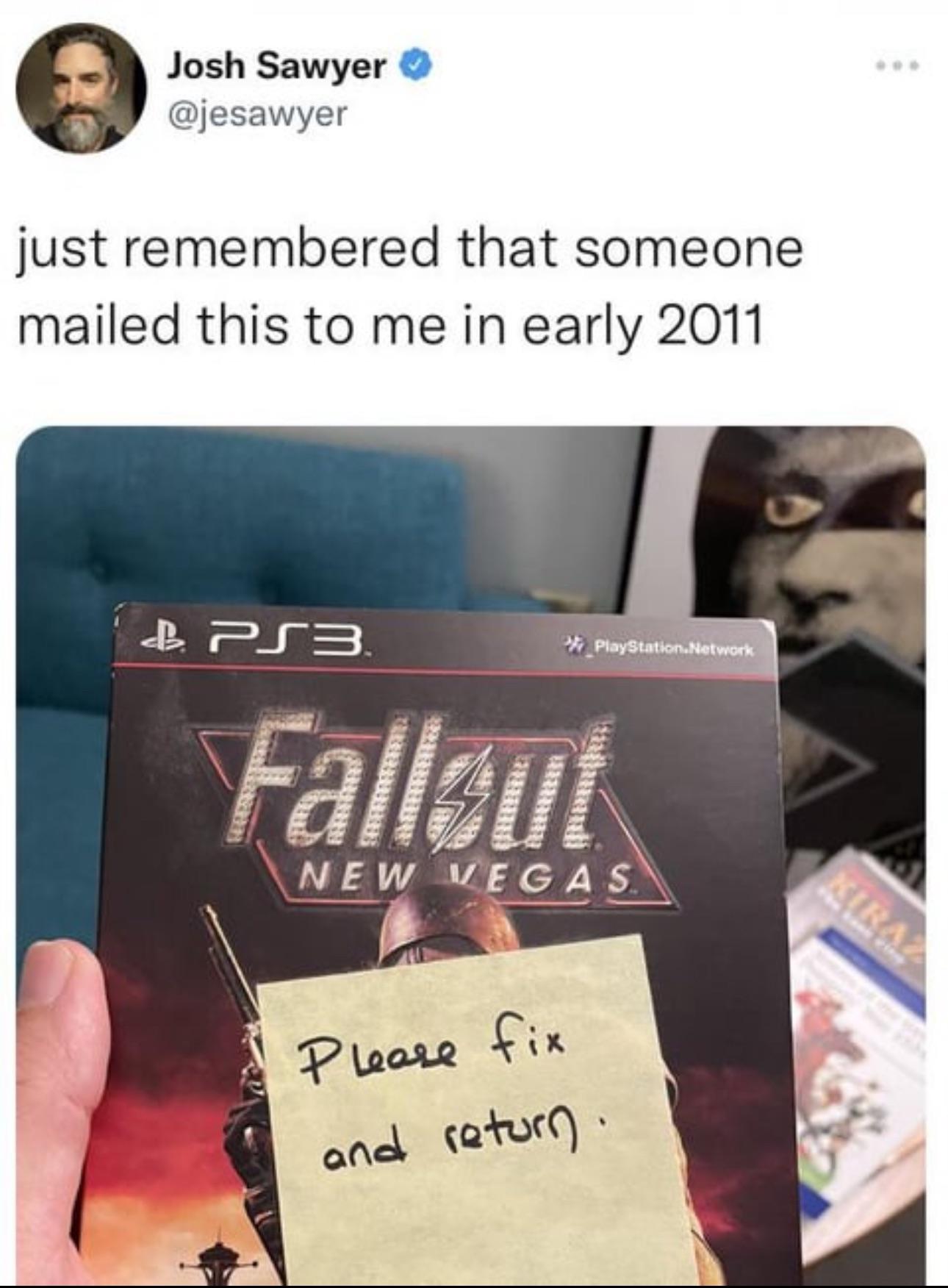
Why Bother?
Look, the default crash reports were okay, but trying to group similar crashes? A total mess. You get slightly different memory addresses, maybe some minor variations in the stack trace, and suddenly you have what looks like a hundred different crashes when it’s really just one or two pesky bugs showing up in slightly different ways. Sorting through that manually was eating up way too much time. I needed a way to quickly fingerprint a crash, to see if it was something new or just the same old ghost haunting the machine.
Hashing the Problem
So, I thought, hashing might be the way to go. Take the important bits of the crash info, hash it down to a single number or string. If the hashes match, chances are high it’s the same underlying issue. I looked around a bit. MD5? SHA? Seemed like overkill, honestly. I didn’t need cryptographic security, I just needed something fast and reasonably unique to act as an identifier.
That’s when I stumbled across FNV, specifically FNV-1a. People kept saying it was simple, really fast, and had decent distribution – meaning less chance of different crashes accidentally getting the same hash. Good enough for me. Didn’t need a cannon to kill a mosquito, you know?
Getting Hands Dirty
First step was actually getting the FNV algorithm working. I found a few implementations online, picked one that looked straightforward. Didn’t want to spend ages writing a hash function myself. Just plugged that into my basic error handling code.
Next, deciding what to hash. This took some trial and error. Just hashing the error message? Not enough context. Hashing the entire stack trace? Too sensitive to minor changes. I settled on a combination:
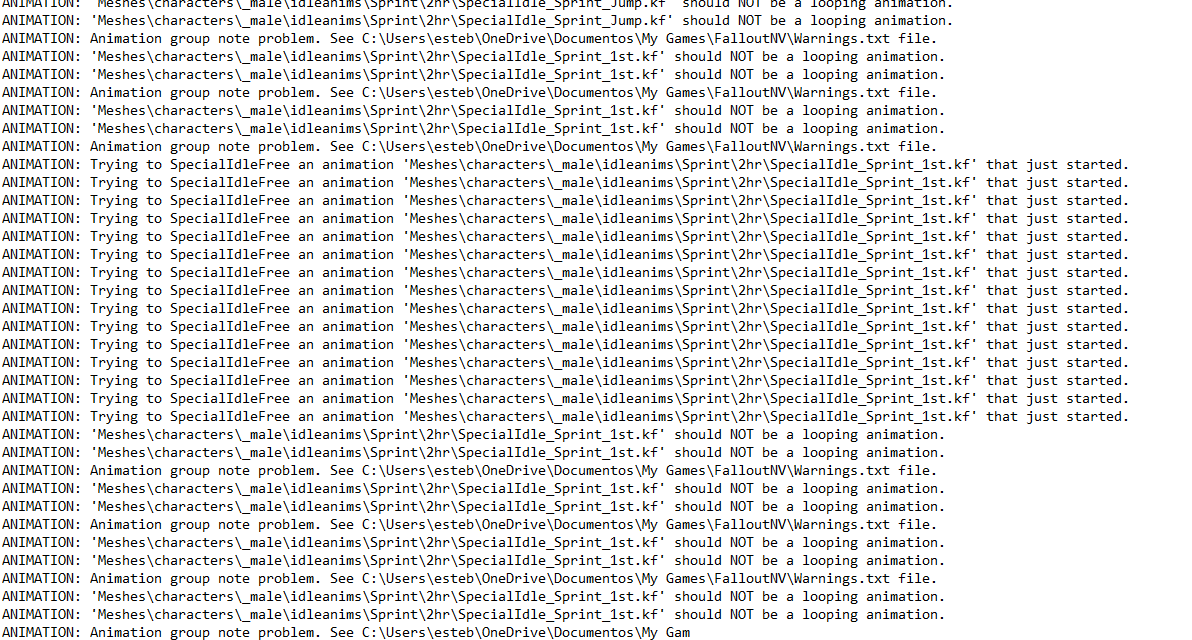
- The exception type or error code.
- The function names from the top, say, 10-15 frames of the call stack. I decided to ignore line numbers and memory addresses because those change all the time for the same logical crash.
- Maybe the module or file where the crash occurred, if available.
The process was simple: when a crash happened, my handler would grab these key pieces of information, string them together, and then feed that whole string into the FNV hash function. Out popped a nice, compact hash value.
Putting It To The Test
Testing was kind of fun, in a morbid way. I started intentionally crashing my test application in various places. I’d trigger the same logical error multiple times and check if the FNV hash came out the same. Mostly, it did, which was the whole point. Then I’d introduce a different bug and verify I got a different hash.
Did I worry about hash collisions? Yeah, a little. FNV isn’t perfect. Two totally different crashes could theoretically produce the same hash. But for the purpose of quick grouping and identification? The chances were low enough to be acceptable. It wasn’t meant to be a foolproof unique ID, just a practical tool to reduce noise. If two different crashes got the same hash, well, I’d eventually figure it out when looking at the details, but it would still group the vast majority correctly.
Fine-Tuning
Initially, I was hashing too much of the stack trace, which made the hash change too often. So I tweaked it to only include the top N frames, focusing on the functions directly involved in the crash. I also made sure to normalize the data a bit before hashing – converting things to lowercase, maybe stripping some standard library paths, just to make the hashes more consistent.
The Outcome
So now, when a crash comes in, it gets tagged with this FNV hash. In my dashboard or log aggregator, I can just group by this hash. Instantly, I see “Oh, crash XYZ happened 500 times today” instead of seeing 500 slightly different reports. It makes prioritizing fixes way easier. Is it perfect? Nah. But it’s a massive improvement over digging through raw crash dumps all day. It was relatively simple to implement and made a real difference in managing incoming issues. Definitely worth the effort.
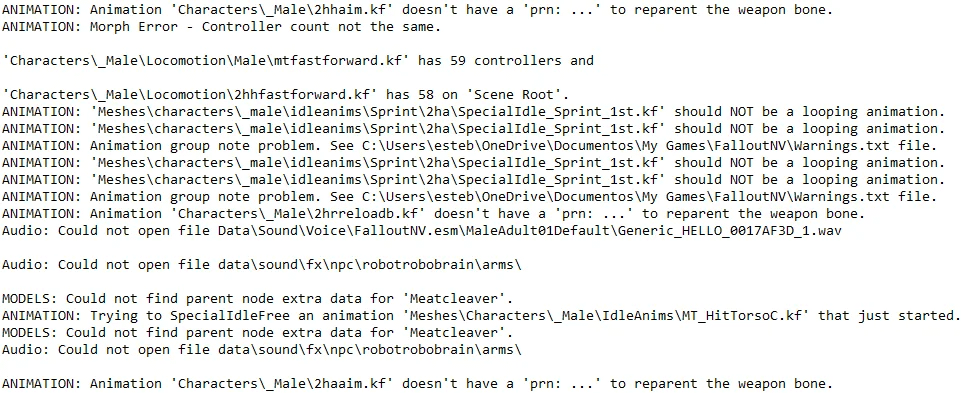