Today, I stumbled upon something called the Firefly algorithm and thought, “Why not give it a shot?” So, I decided to mess around with planar sets in Firefly. Let me tell you, it was a bit of a rollercoaster.
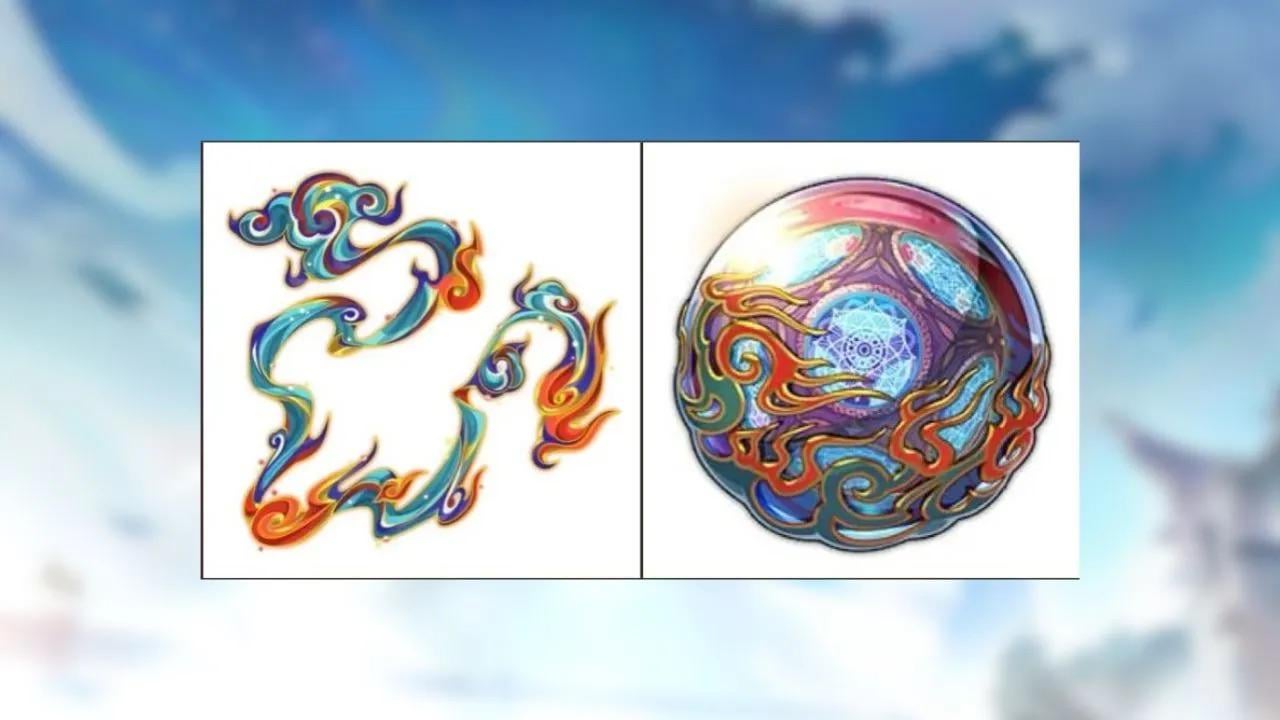
Getting Started
First things first, I had to get my hands dirty with some coding. I fired up my old code editor – the one I hadn’t used in ages – and started typing away. I chose Python because, well, it’s pretty straightforward for this kind of thing.
- Set up the environment: Made sure Python was installed. It was already there, phew!
- Import necessary libraries: I needed
NumPy
for the math stuff andMatplotlib
for plotting.
I found some basic Firefly algorithm implementation online and tweaked it a bit. It wasn’t perfect, but hey, it was a start.
Diving into the Code
The main idea was to represent the planar set as a bunch of points and then use the Firefly algorithm to find, I don’t know, the best arrangement? I was really just feeling it out as I went.
Here’s a rough idea of what I did:
- Initialize fireflies: Randomly placed some points (fireflies) on a 2D plane.
- Calculate brightness: This was supposed to represent how good a firefly’s position was. I just used the distance from the origin as a simple measure.
- Move fireflies: The core of the algorithm. Each firefly moves towards brighter ones, with some randomness thrown in.
I ran into a bunch of issues here. Sometimes the fireflies just clumped together in one spot, and other times they just went flying off the screen. Debugging this was like finding a needle in a haystack – frustrating and time-consuming.
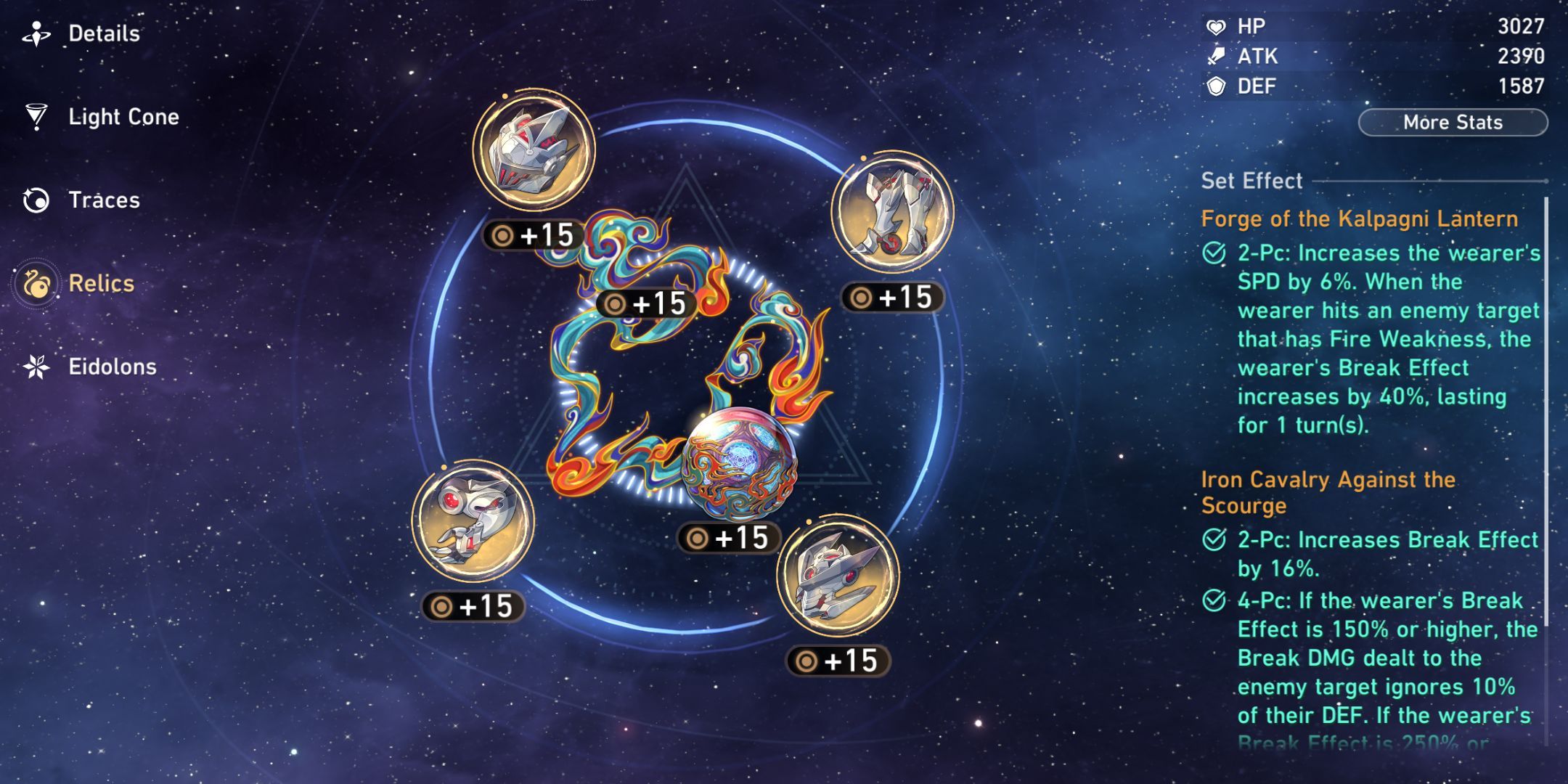
Tweaking and Tuning
After hours of fiddling with parameters, I started to get some interesting results. I played around with the number of fireflies, the randomness factor, and the brightness calculation. Each change brought something new to the table, and it was pretty cool to watch.
- Number of fireflies: Too few, and it wasn’t interesting. Too many, and my computer started to lag. I found 20 to be a good middle ground.
- Randomness factor: This was a tricky one. Too much randomness, and the fireflies just wandered aimlessly. Too little, and they all ended up in the same place.
- Brightness calculation: I tried different formulas here, like distance to a specific point or even just a random value to see what would happen.
I probably spent way too much time on this, but it was kind of addictive.
Final Results
In the end, I managed to get some pretty neat patterns. The fireflies would start all spread out and then slowly move towards each other, forming interesting shapes and clusters. It wasn’t exactly what I expected, but it was definitely something.
Here are some of the things I noticed:
- The fireflies tended to form clusters around the areas I defined as “brighter.”
- Sometimes, a few fireflies would get left behind, creating a kind of scattered effect.
- The final arrangement was always different, even with the same settings.
It was a fun experiment, and I learned a lot about the Firefly algorithm and how it can be used to play with planar sets. Sure, it was messy, and I probably made a ton of mistakes, but that’s how you learn, right?
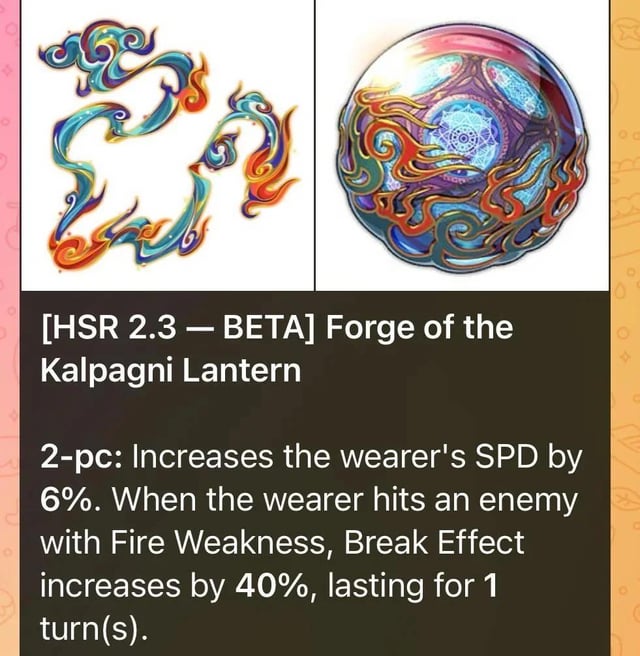
So, yeah, that was my adventure with the Firefly algorithm and planar sets. If you’re looking for something to do on a rainy day, give it a try. Just be prepared to lose a few hours – or maybe even a whole day – tweaking and staring at your screen.