Okay, so I wanted to add a cool countdown timer to a banner on my website. I’m working on this “Robin” project, and I figured a countdown would be a neat way to show, like, when the next big update is dropping. I’ve seen these on other sites, and they always seem to grab my attention.
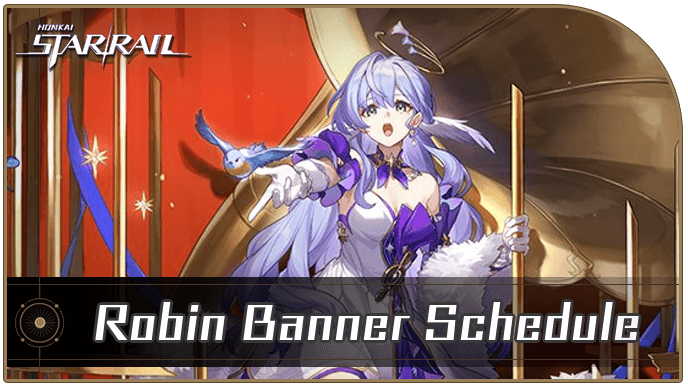
First, I gotta say, I’m not a coding wizard. I know my way around HTML and CSS, and I can fumble through some basic JavaScript, but that’s about it. So, I knew I’d need some help along the way. I started by just messing around with some HTML to get the basic layout.
I created a simple div to hold the banner, and inside that, I put some elements for the days, hours, minutes, and seconds. Just plain text at first, nothing fancy. Something like this:
Time Left:
Days,
Hours,
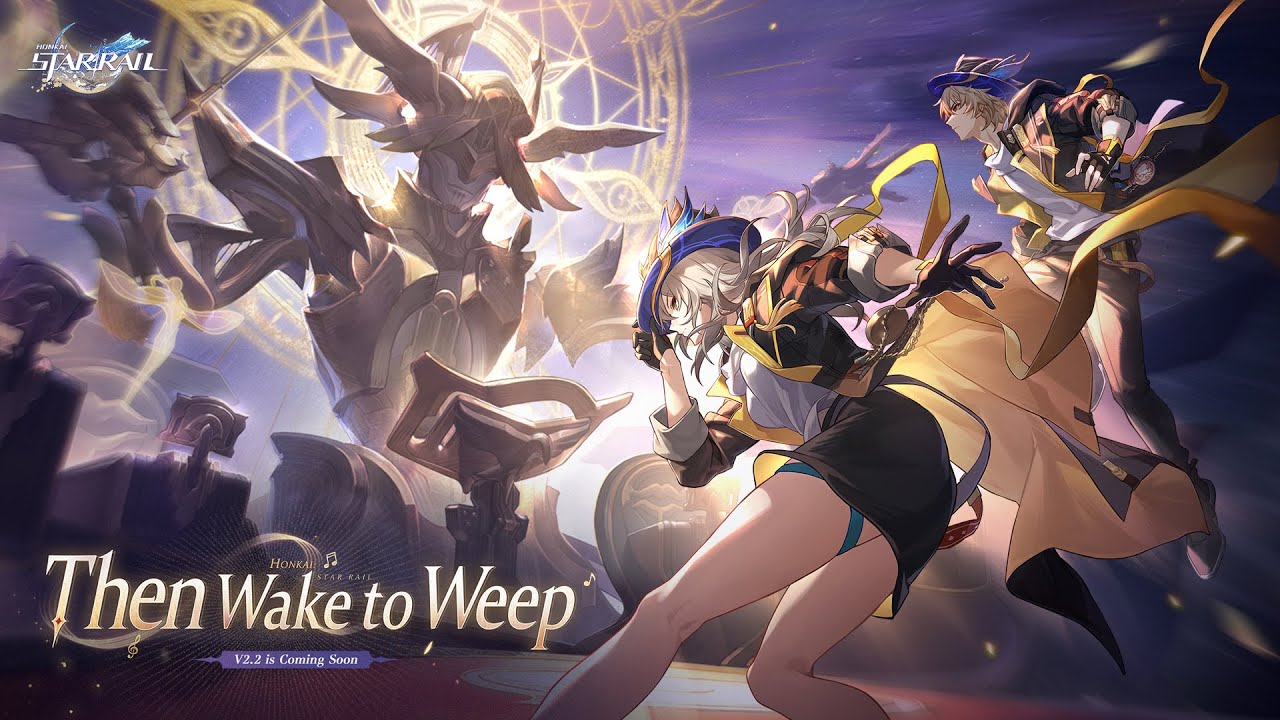
Minutes,
Seconds
Next, I needed to make it actually count down. This is where the JavaScript comes in, and where I definitely needed to do some Googling. I found a bunch of countdown scripts, but most of them were way more complicated than I needed. I just wanted something simple and straightforward.
I ended up finding a basic script and tweaking it. Basically, it works by getting the current date and time, and then calculating the difference between that and the target date (the date I want the countdown to end). Then, it breaks that difference down into days, hours, minutes, and seconds.
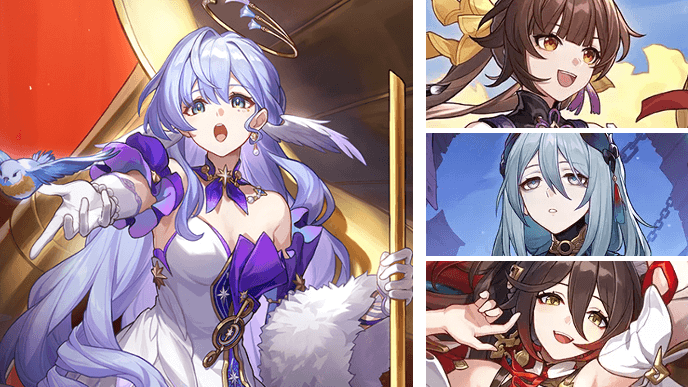
Here is the Javascript I ended up using:
javascript
// Set the date we’re counting down to
var countDownDate = new Date(“Jan 5, 2025 15:37:25”).getTime();
// Update the count down every 1 second
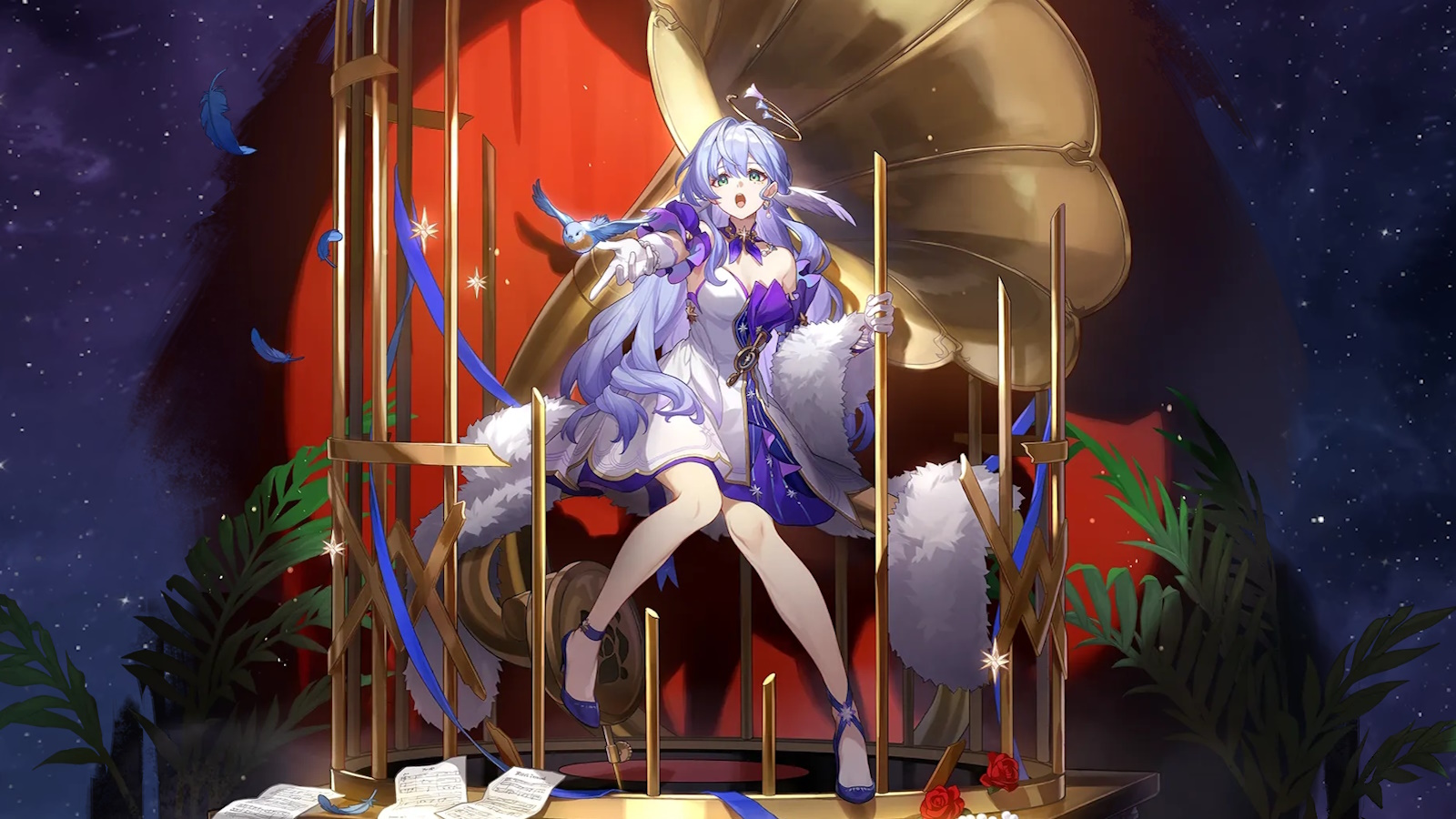
var x = setInterval(function() {
// Get today’s date and time
var now = new Date().getTime();
// Find the distance between now and the count down date
var distance = countDownDate – now;
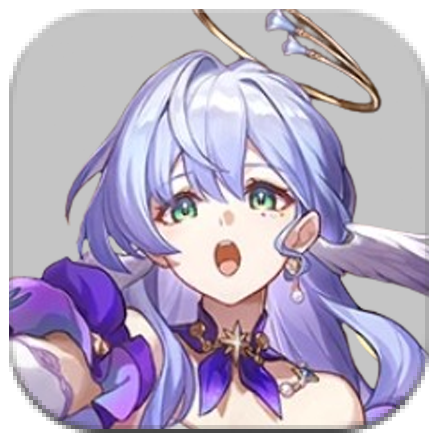
// Time calculations for days, hours, minutes and seconds
var days = *(distance / (1000 60 60 24));
var hours = *((distance % (1000 60 60 24)) / (1000 60 60));
var minutes = *((distance % (1000 60 60)) / (1000 60));
var seconds = *((distance % (1000 60)) / 1000);
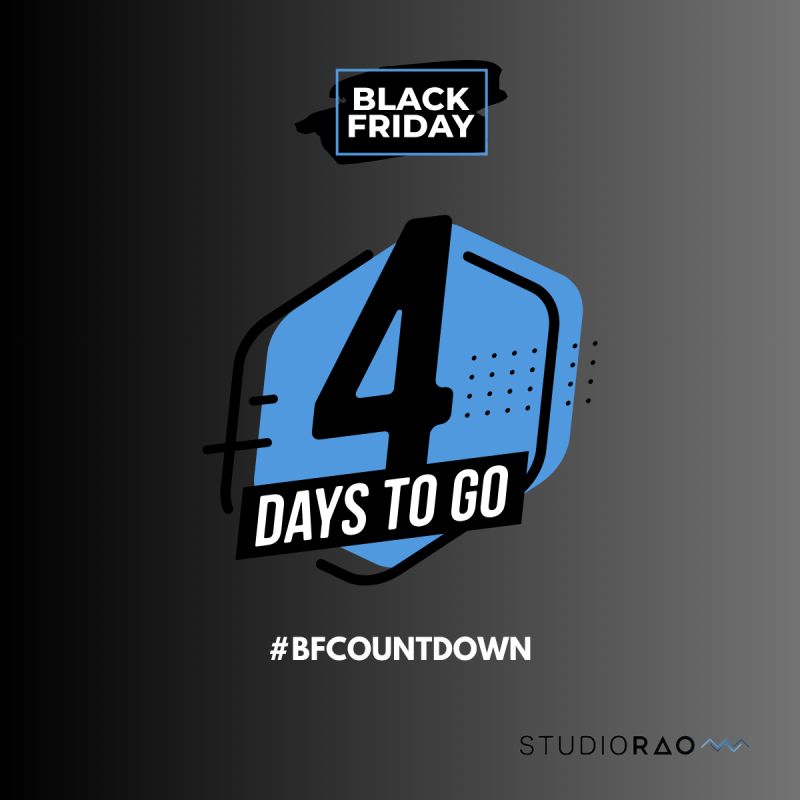
// Display the result in the element with id=”demo”
*(“days”).innerHTML = days;
*(“hours”).innerHTML = hours;
*(“minutes”).innerHTML = minutes ;
*(“seconds”).innerHTML = seconds;
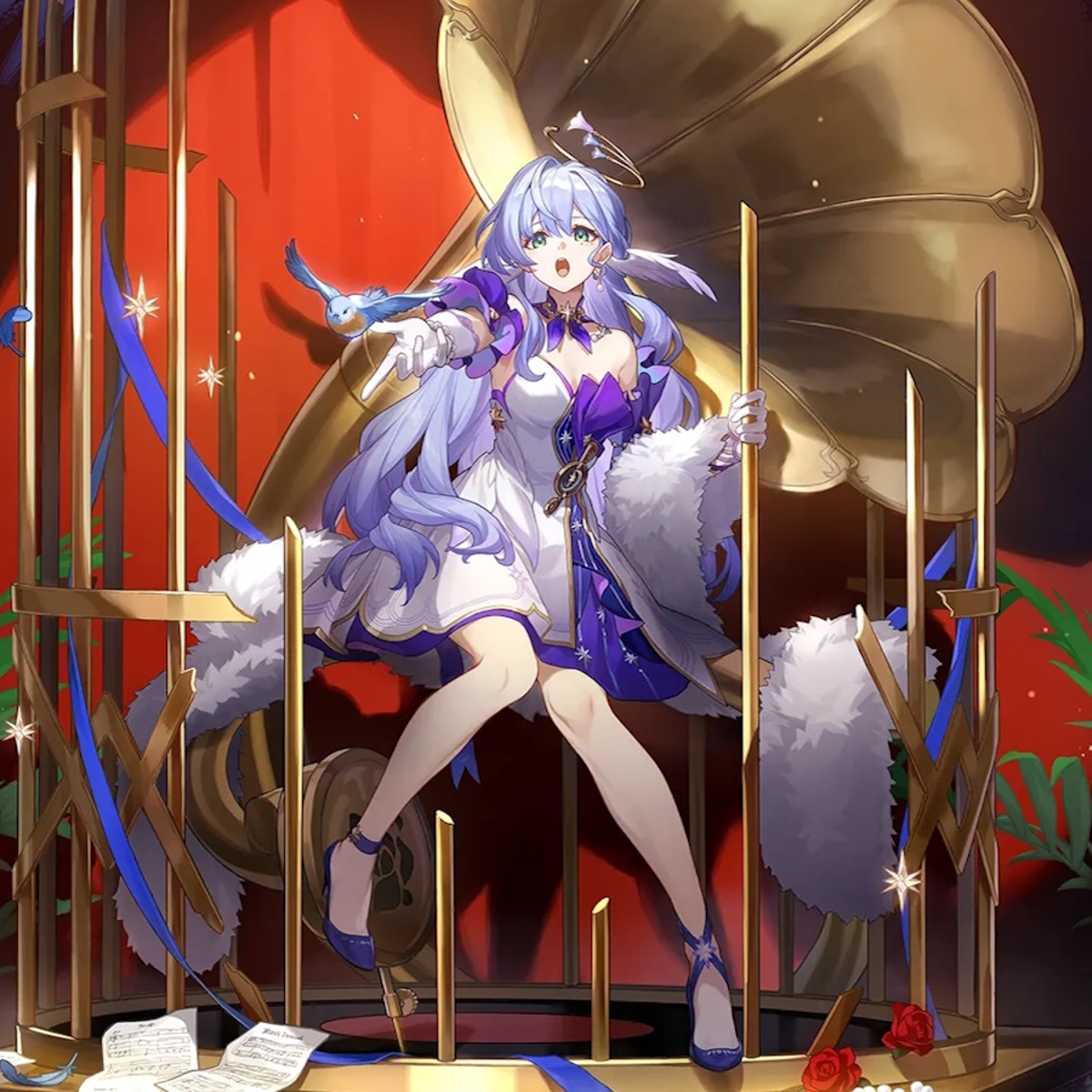
// If the count down is finished, write some text
if (distance < 0) {
clearInterval(x);
*(“demo”).innerHTML = “EXPIRED”;
}, 1000);
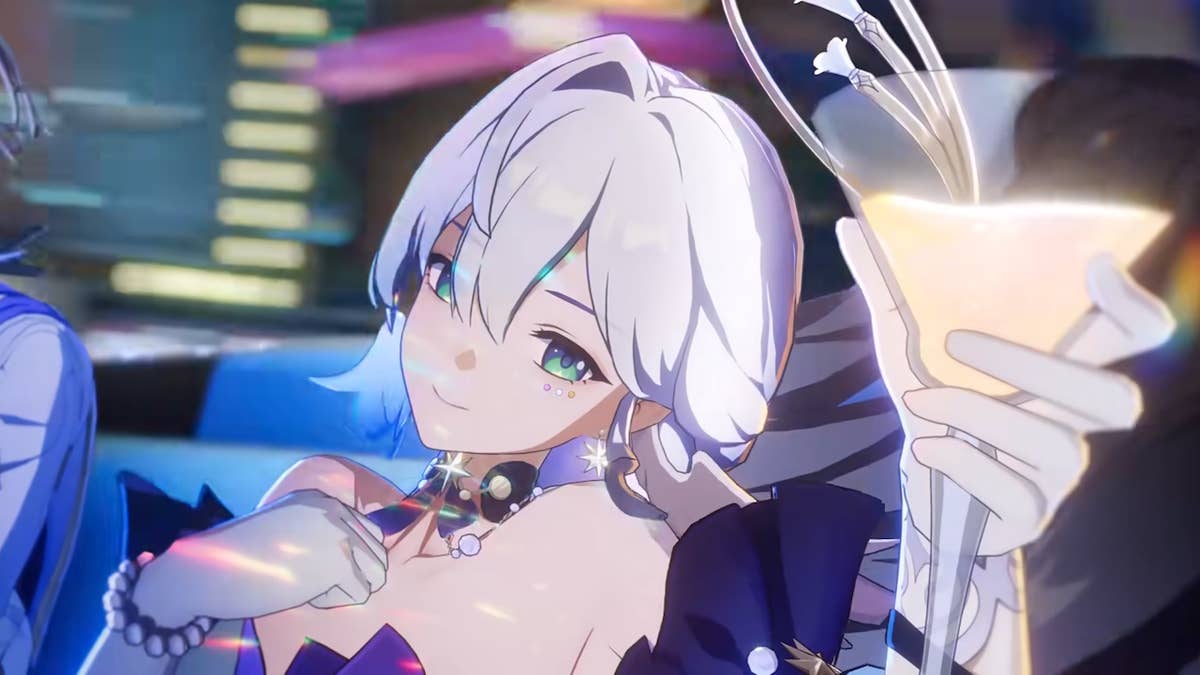
It uses a setInterval function to update the countdown every second. Inside that function, it does the calculations and then updates the HTML elements with the new values. I had to make sure the IDs in the JavaScript matched the IDs in my HTML, otherwise, it wouldn’t work. Also, I need put my end date.
Finally, I added some simple CSS to style the banner and the timer. I just gave it a background color, some padding, and made the timer numbers a bit bigger and bolder. Nothing too crazy, just enough to make it look decent.
It took a bit of trial and error, but I finally got it working! It’s not the most sophisticated countdown timer in the world, but it does the job. And, honestly, I’m pretty proud of myself for figuring it out, especially with my limited coding skills. Now I just got to keep on my Robin project to make it on time!